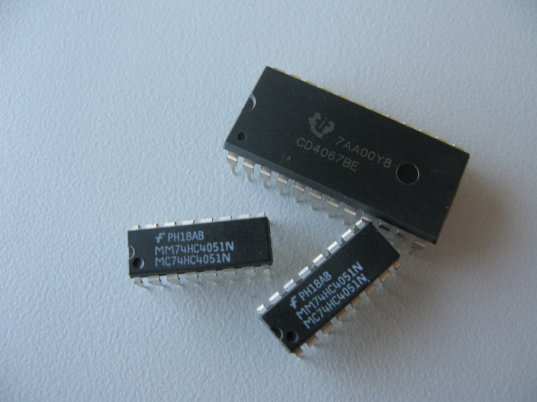
Shift Registers and Multiplexers are integrated components (IC) which allow one to control multiple inputs and outputs from a microcontroller i.e. Arduino. Multiplexers are useful in that they can handle both digital and analog input and output – compared to a Shift Register. A multiplexer has several inputs and one output or vice versa, and the majority of multiplexers are bi-directional. Any of the multiplexer inputs can be routed to the output – although, only one at a time. Whereas, with a Shift Register the digital outputs could be managed in parallel.
Multiplexers have a series of address pins, and when these are set the input is routed to the output. The address of the channel you would like to access is deposited on the address pins as a binary number i.e. Address 000 = channel 0 or Address 001 = Channel 1.
An 8 channel multiplexer will provide 3 address pins based on binary to access each channel. Each address pin has two states either 0 or 1, so we have 2³ options, which becomes 2 x 2 x 2 = 8 channels.
/* Binary Array - Count 0 to 8 */ // Channels 0 1 2 3 4 5 6 7 int bin [] = {000, 1, 10, 11, 100, 101, 110, 111};
Now that we have defined the channel number binary equivalents, we would need to write these values to the address pins – one after the other – to access that particular channel.
In the example below, we poll each channel of the multiplexer (from within the for loop construct) and then momentarily set each output low (0) before moving to the next output channel.
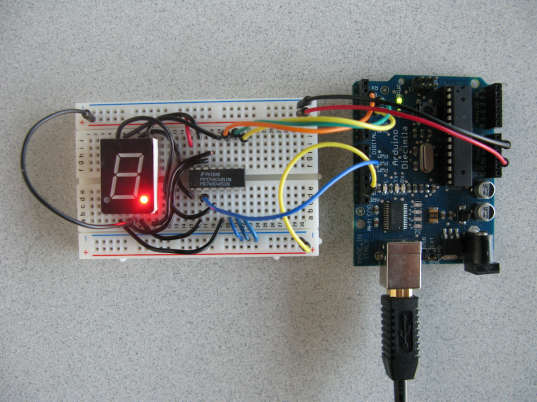
/* Multiplexer Wiring Schematic - Part Number MC74HC4051N +5V-R1-(.)-01 -| |- VCC-+5V +5V-R1-(.)-03 -| |- 02-(.)-R1-+5V +5V-R1-(A0)-| |- 04-(.)-R1-+5V +5V-R1-(.)-07 -| |- 08-(.)-R1-+5V +5V-R1-(.)-05 -| |- 06-(.)-R1-+5V 0V-INH -| |- A (D2) 0V-VEE -| |- B (D3) 0V-VSS -| |- C (D4) Key Map: (.) = Connection Point To Multiplexer (A0) = Arduino Analog Pin 0 (D2), (D3), (D4) = Arduino Digital Pin 2, 3 and 4 +5V = Power 0V = GND */ // Constants #define MULTIPLEXER_S0 2 #define MULTIPLEXER_S1 3 #define MULTIPLEXER_S2 4 // Declare Multiplexer Access Variabless int pinA, pinB, pinC, pinABC; void setup() { //Serial.begin(9600); // Set MULTIPLEXER_S0/1/2 Mode pinMode(MULTIPLEXER_S0, OUTPUT); pinMode(MULTIPLEXER_S1, OUTPUT); pinMode(MULTIPLEXER_S2, OUTPUT); // Initialise Multiplexer Access Variabless pinA = pinB = pinC = pinABC = 0; } void writeToMultiplexer(int pot) { pinABC = pot; pinA = pinABC & 0x01; pinB = (pinABC >> 1) & 0x01; pinC = (pinABC >> 2) & 0x01; // Poll Multiplexer digitalWrite(MULTIPLEXER_S0, pinC); digitalWrite(MULTIPLEXER_S1, pinB); digitalWrite(MULTIPLEXER_S2, pinA); // Digital Output //pinMode(11, OUTPUT); //digitalWrite(11, 0); analogWrite(11, 0); } // End of writeToMultiplexer() int getAnalog() { int getAnalog = analogRead(0)/128; //Serial.println(getAnalog); return getAnalog; } void loop () { writeToMultiplexer(getAnalog()); delay(25); } // End LoopUnless otherwise stated, this code is released under the MIT License – Please use, change and share it.
In the example below, the Arduino polls a series of pins on the multiplexer to create one Hex Decimal character at a time.
/* Multiplexer Wiring Schematic - Part Number MC74HC4051N +5V-R1-(.)-01 -| |- VCC-+5V +5V-R1-(.)-03 -| |- 02-(.)-R1-+5V +5V-R1-(A0)-| |- 04-(.)-R1-+5V +5V-R1-(.)-07 -| |- 08-(.)-R1-+5V +5V-R1-(.)-05 -| |- 06-(.)-R1-+5V 0V-INH -| |- A (D2) 0V-VEE -| |- B (D3) 0V-VSS -| |- C (D4) Key Map: (.) = Connection Point To Multiplexer (A0) = Arduino Analog Pin 0 (D2), (D3), (D4) = Arduino Digital Pin 2, 3 and 4 +5V = Power 0V = GND */ // Constants #define MULTIPLEXER_S0 2 #define MULTIPLEXER_S1 3 #define MULTIPLEXER_S2 4 // Declare Multiplexer Access Variabless int pinA, pinB, pinC, pinABC; // Hex Decimal Characters 0 - F int numArray[16][7] = { { 1, 2, 3, 4, 5, 6 }, // 0 { 2, 3 }, // 1 { 1, 2, 4, 5, 7}, // 2 { 1, 2, 3, 4, 7}, // 3 { 2, 3, 6, 7}, // 4 { 1, 3, 4, 6, 7}, // 5 { 1, 3, 4, 5, 6, 7}, // 6 { 1, 2, 3}, // 7 { 1, 2, 3, 4, 5, 6, 7},// 8 { 1, 2, 3, 4, 6, 7}, // 9 { 1, 2, 3, 5, 6, 7}, // A { 1, 2, 3, 4, 5, 6, 7},// B { 1, 4, 5, 6}, // C { 1, 2, 3, 4, 5, 6}, // D { 1, 4, 5, 6, 7}, // E { 1, 5, 6, 7} // F }; int numLen[16] = {6, 2, 5, 5, 4, 5, 6, 3, 7, 6, 6, 7, 4, 6, 5, 4}; void setup() { //Serial.begin(9600); // Set MULTIPLEXER_S0/1/2 Mode pinMode(MULTIPLEXER_S0, OUTPUT); pinMode(MULTIPLEXER_S1, OUTPUT); pinMode(MULTIPLEXER_S2, OUTPUT); // Initialise Multiplexer Access Variabless pinA = pinB = pinC = pinABC = 0; } void writeToMultiplexer(int pot) { // Iterate through all 8 Pins of the Multiplexer for (int i = 0; i < numLen[pot]; i++) { // Binary Access to Input Pins pinABC = numArray[pot][i]; pinA = pinABC & 0x01; pinB = (pinABC >> 1) & 0x01; pinC = (pinABC >> 2) & 0x01; // Poll Multiplexer digitalWrite(MULTIPLEXER_S0, pinC); digitalWrite(MULTIPLEXER_S1, pinB); digitalWrite(MULTIPLEXER_S2, pinA); analogWrite(11, 0); delay(20); } } // End of writeToMultiplexer() int getAnalog() { int getAnalog = analogRead(0)/64; //Serial.println(getAnalog); return getAnalog; } void loop () { writeToMultiplexer(getAnalog()); delay(25); } // End LoopUnless otherwise stated, this code is released under the MIT License – Please use, change and share it.
In the example below, the Arduino polls each pin on the multiplexer – until it the analog input surpasses the defined threshold.
/* Multiplexer Wiring Schematic - Part Number MC74HC4051N +5V-R1-(.)-01 -| |- VCC-+5V +5V-R1-(.)-03 -| |- 02-(.)-R1-+5V +5V-R1-(A0)-| |- 04-(.)-R1-+5V +5V-R1-(.)-07 -| |- 08-(.)-R1-+5V +5V-R1-(.)-05 -| |- 06-(.)-R1-+5V 0V-INH -| |- A (D2) 0V-VEE -| |- B (D3) 0V-VSS -| |- C (D4) Key Map: R1 = 10K Ohm Resistor Connected to +5V (VCC) or GND C1 = 0.1microFarads Connected to 0V (GND) (.) = Connection Point To Multiplexer (A0) = Arduino Analog Pin 0 (D2), (D3), (D4) = Arduino Digital Pin 2, 3 and 4 +5V = Power 0V = GND */ // Constants #define MULTIPLEXER_S0 2 #define MULTIPLEXER_S1 3 #define MULTIPLEXER_S2 4 #define ANALOG_PIN_READ 0 #define THRESHOLD 768 // Declare Multiplexer Access Variabless int pinA, pinB, pinC, pinABC; // Binary Array - Count 0 to 8 int bin [] = {000, 1, 10, 11, 100, 101, 110, 111}; // Control Boolean boolean cont = true; // Continue Polling the Multiplexer boolean isHigh = false; // int i; // Pin Counter 0 to 7 void setup() { // Set MULTIPLEXER_S0/1/2 Mode pinMode(MULTIPLEXER_S0, OUTPUT); pinMode(MULTIPLEXER_S1, OUTPUT); pinMode(MULTIPLEXER_S2, OUTPUT); // Initialise Multiplexer Access Variabless pinA = pinB = pinC = pinABC = 0; } void samplePin(int a, int b, int c, int pinNum, int mulNum) { boolean isOn = true; int declareOnOff = -1; while (isOn) { // Poll Multiplexer digitalWrite(MULTIPLEXER_S0, pinC); digitalWrite(MULTIPLEXER_S1, pinB); digitalWrite(MULTIPLEXER_S2, pinA); /* * if (analogRead(mulNum) >= THRESHOLD) * { * declareOnOff = 1; * Your code Here * delay(100); * } * else * { * declareOnOff = 0; * Your Code Here * isOn = false; * isHigh = false; * cont = true; * } */ if (analogRead(mulNum) >= THRESHOLD) { declareOnOff = 1; analogWrite(11, 200); delay(100); } else { declareOnOff = 0; analogWrite(11, 0); // Return isOn = false; isHigh = false; cont = true; } } // End While (isOn) } // End samplePin void readFromMultiplexer() { while (cont) { i = 0; // Controls access to Multiplex Pins while ((!(isHigh)) && (i <= 7)) { /* Binary Access to Input Pins More details about why we convert to Binary */ pinABC = bin[i]; pinA = pinABC & 0x01; pinB = (pinABC>>1) & 0x01; pinC = (pinABC>>2) & 0x01; // Poll Multiplexers digitalWrite(MULTIPLEXER_S0, pinC); digitalWrite(MULTIPLEXER_S1, pinB); digitalWrite(MULTIPLEXER_S2, pinA); // MultiPlexer - Analog Pin 0 - Pin 2 if (analogRead(ANALOG_PIN_READ) >= THRESHOLD) { isHigh = true; cont = false; samplePin(pinC, pinB, pinA, i, ANALOG_PIN_READ); } else { i++; } } // End While ((!(isHigh)) && (i <= 7)) } // End While(cont) } // End of writeToMultiplexer() void loop () { readFromMultiplexer(); delay(25); } // End LoopUnless otherwise stated, this code is released under the MIT License – Please use, change and share it.