SERIAL COMMUNICATION
This tutorial covers the topic of Serial Communication between the Arduino and Max/MSP. The “serial” in Serial Communication means that the data is transferred one byte (8 bits) at a time, and is immediately followed by another byte until the data transfer is completed. Arduino and Max/MSP communicate via a serial line over USB.
The tutorial Max/MSP patch and accompanying Arduino code has been implemented to showcase the data transfer possibilities between Max/MSP and Arduino. The Max/MSP patch contains several sub-patches: Serial Config, Analog In, Digital In, Digital Out and Analog Out.
In example below, the Arduino does not read any sensor data – it waits for a message from the host computer and once received it begins a serial response.
int serialMessage = -1; // Message Received Flag int messageReceived = 0; // Send Random Value to Host long rnd = 0; void setup() { // Set the serial baudrate, used internally by arduino Serial.begin(9600); } void loop() { if (Serial.available()) { serialMessage = Serial.read(); Serial.flush(); // Clear Serial Incoming Buffer messageReceived = 1; } if (messageReceived) { rnd = random(1024); Serial.print(rnd ); // Print rnd value Serial.print(" "); // Print Space Char Serial.println(); // Print LineFeed delay(50); // Pause } }Unless otherwise stated, this code is released under the MIT License – Please use, change and share it.
The Arduino opens a serial port connection at 9600 BAUD – this is the speed at which Arduino will communicate with Max/MSP and vice versa. If the data appears mangled or misrepresented, check that both the Arduino and Max/MSP are set to communicate at the same BAUD rate.
The Max/MSP serial object, as seen below, is used to communicate with generic serial devices that do not require additional hardware in order to operate i.e. MIDI. The serial ports in Max/MSP are are labeled (a, b, c etc.), to discover your available serial ports click on the “print” and a list of ports will appear in the Max Window. To change serial port, unlock the patcher (ctrl-e or apple-e) and enter the desired letter into the serial object.
The serial object also takes a number of arguments to set up the communications channel. The second argument sets the BAUD rate and needs to match that of the Arduino. There are some additional arguments that can be set in the serial object: Data Bits, Stop Bits and Parity. As with the Arduino, the Max/MSP serial object can transmit and receive serial data.
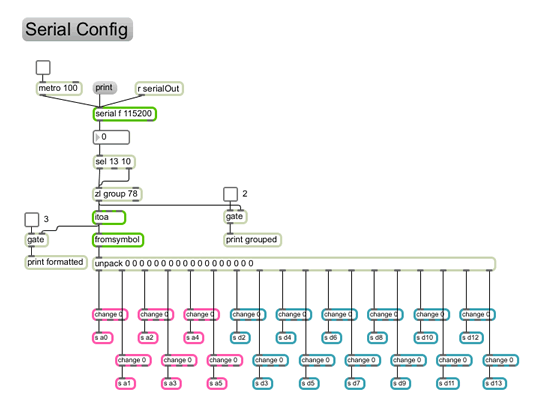
Once you have the Arduino and Max/MSP setup, it is worth noting that the Arduino transmits its serial data as ASCII. For example, when Arduino transmits the number 15 it is represented as ASCII value of 49 (“1”) and 53 (“5”), and a Space character is represented as 32. In Max/MSP, the Serial Config patch has an option which allows you to view the raw data. Useful, when debugging serial communications.
As mentioned earlier, the Max/MSP tutorial patch has been split into specific areas of interest with respect to the Arduino – Max/MSP communication channel. These sub-patches operate independently of each other. The Analog In and Digital In sub-patches poll the Arduino every 100ms, each time sending a series of data from the Arduino analog/digital pins to Max/MSP. The Analog Out and Digital Out sends a series of data which the Arduino acts upon.
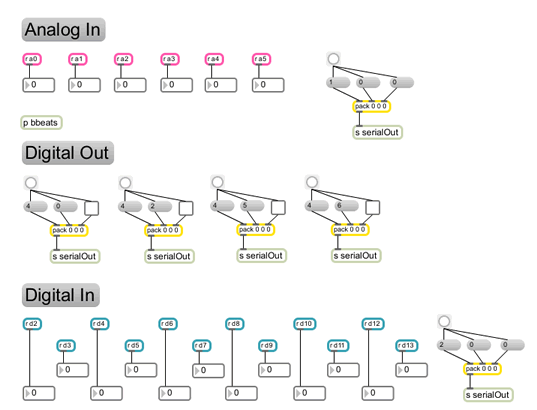
References & Tutorial Files:
Cycling 74: Makers of Max/MSP & Jitter.
Arduino: Technical Data regarding Arduino.
Ladyada: Arduino Information & HowTo’s.The Max/MSP patch was created using Max/MSP v5.04. The Sensor Lab has Max/MSP v5.04, Arduino to Max Tutorial Files.